Some collections can be searched, based on the fields they include. You can include the X-Filter
header to pass in JSON-formatted filter objects. For example, here's a request to list Linode types that use our standard
class:
curl "https://api.linode.com/v4/linode/types" \
-H 'X-Filter: { "class": "standard" }'
The filter's keys are from the object you're filtering, using that object's accepted values.
What's filterable
You can determine what's filterable in various ways:
- Review the operation's response description. If a parameter is labeled as Filterable in its description in the RESPONSES section, you can include it in the
X-Filter
header.
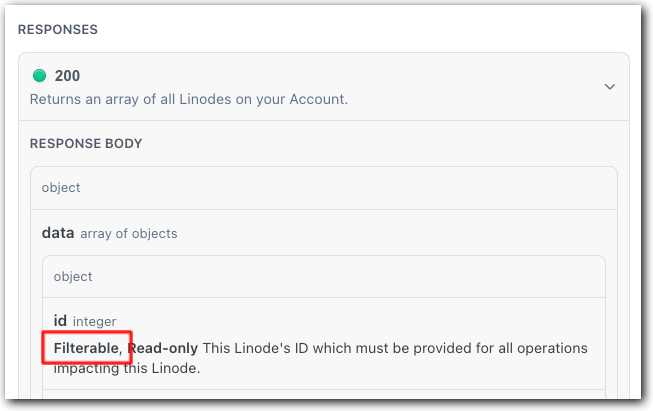
- Review the API specification. If a parameter is marked as
x-linode-filterable: true
in the API specification, it can be included in theX-Filter
header.
Nested parameters and filtering
If a parameter is labeled as Filterable, but it exists as a child of another parameter, use dot notation to represent both the parent and child parameters. For example, lets say a parent parameter is resource
and it has a child parameter type
that's labeled as filterable:
"resource": {
"id": 3456,
"type": "widget", <== This can be filtered
}
The call to filter on that parameter would look like this:
curl "https://api.linode.com/v4/linode/resources" \
-H 'X-Filter: { "resource.type": "widget" }'
Filter operators
You can also add multiple filters by including more than one key. For example, here's how to filter for standard
Linode types that offer one vcpu
:
curl "https://api.linode.com/v4/linode/types" \
-H 'X-Filter: { "class": "standard", "vcpus": 1 }'
This filter automatically applies AND
logic as a filter operator. So, all conditions need to be met to display a filtered response.
You can also set the response to display either Linode types using the standard
class, or Linode types with one vcpu
. In this case, you need to add the +or
filter operator:
curl "https://api.linode.com/v4/linode/types" \
-H 'X-Filter: { "+or": [ { "vcpus": 1 }, { "class": "standard" } ] }'
Each filter in the +or
array is its own filter object, and all conditions in it use AND
logic, as they were in the previous example.
Supported filter operators
Operators form the keys of a filter object. Their value needs to be of the appropriate type, and they're evaluated as follows:
OPERATOR | TYPE | DESCRIPTION |
---|---|---|
+and | array | All conditions need to be true. |
+or | array | At least one condition needs to be true. |
+gt | number | The value needs to be greater than the provided number. |
+gte | number | The value needs to be greater than or equal to the provided number. |
+lt | number | The value needs to be less than the provided number. |
+lte | number | The value needs to be less than or equal to the provided number. |
+contains | string | The provided string needs to be in the value. |
+neq | string | The provided string is left out of the results. |
+order_by | string | Order results based on the provided attribute. The attribute needs to be filterable. |
+order | string | Sort in ascending (asc ) or descending (desc ) order. This defaults to asc . Requires +order_by . |
Here's another example of listing Linode types and filtering the response to those with memory equal to or higher than 61440:
curl "https://api.linode.com/v4/linode/types" \
-H '
X-Filter: {
"memory": {
"+gte": 61440
}
}'
You can combine and nest operators to construct arbitrarily complex queries. This example uses the same operation to list all Linode types that are either standard
or highmem
class, or have between 12 and 20 VCPUs:
curl "https://api.linode.com/v4/linode/types" \
-H '
X-Filter: {
"+or": [
{
"+or": [
{
"class": "standard"
},
{
"class": "highmem"
}
]
},
{
"+and": [
{
"vcpus": {
"+gte": 12
}
},
{
"vcpus": {
"+lte": 20
}
}
]
}
]
}'