Redirect pages based on location
In this tutorial you'll learn how to create an EdgeWorkers function that provides a customized user experience. Using less than 35 lines of code and EdgeScape geo data you can redirect web-site visitors to country specific content.
This example invokes an EdgeWorkers script at the onClientRequest
phase of an HTTP request as the first event before caching occurs. Then, based on the request's country of origin, the response redirects users to location specific content.
You can find the redirect-geo code sample in the EdgeWorkers examples GitHub repo.
Before you begin
Before you can configure your EdgeWorkers function, you need to do a few things:
-
Get access to the EdgeWorkers interface in Akamai Control Center. This interface lets you perform the administrative tasks required to execute EdgeWorkers functions. You need to have a Control Center account that's been granted access to the EdgeWorkers Management application. If you're not sure, talk to your local Akamai admin, or contact your Akamai account team.
-
Add EdgeWorkers to your contract. If you have an active Akamai contract and login credentials for Akamai Control Center you can sign up for the EdgeWorkers Evaluation tier. The Evaluation tier lets you try EdgeWorkers for free and without a time limit. If you're not already an Akamai customer or you don't have access to Akamai Control Center you can sign up for an EdgeWorkers free trial.
1. Create an EdgeWorker ID
An EdgeWorker ID lets you enable the EdgeWorkers behavior in Akamai Control Center. It's also a unique identifier for your EdgeWorkers code.
-
Log in to Control Center.
-
Go to ☰ > CDN > EdgeWorkers.
-
Click Create EdgeWorker ID.
You cannot edit this auto-generated, unique identifier.
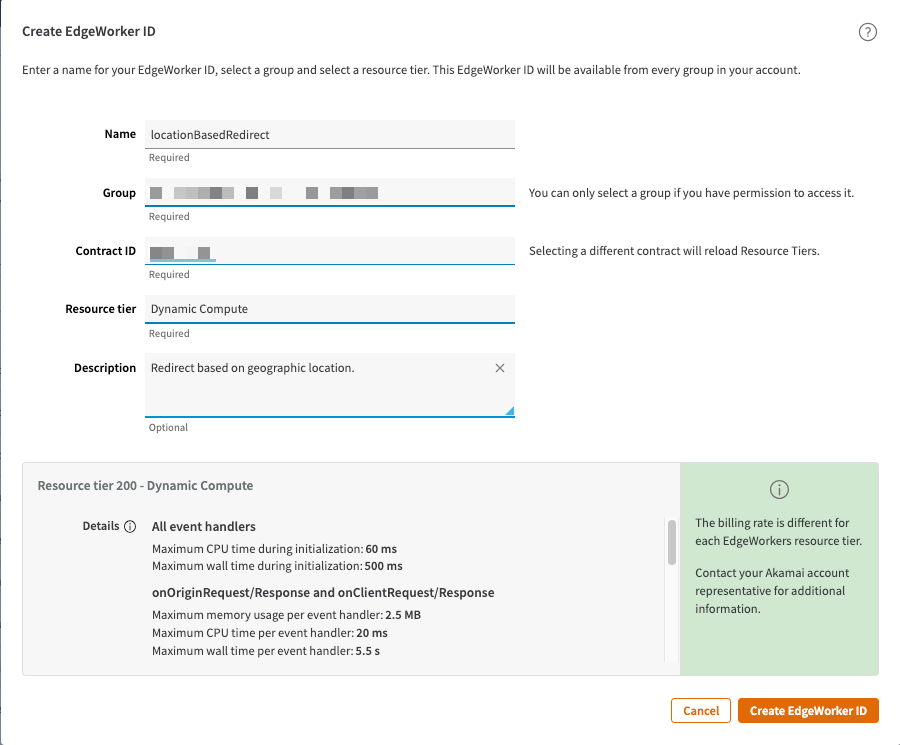
-
Enter
Geolocation redirect
as the name for the EdgeWorker ID. -
Select a Group Association.
You can select only a group that you have permission to access.
-
Select a Contract ID to associate with the EdgeWorker ID.
The available resource tiers are filtered based on the contract ID you select.
The Contract ID field only appears if you have more than one contract ID associated with your account.
-
Select a resource tier.
EdgeWorkers resource tiers currently include and Dynamic Compute, Enterprise Compute, and Basic Compute.
The limits for each resource tier are different. You can view the limits for the selected resource tier in the details section or you can view the Resource tier limitations section.
-
Click Create EdgeWorker ID.
2. Add the EdgeWorkers behavior
When you add the EdgeWorkers behavior in Property Manager you can also define which requests apply EdgeWorkers functions. By limiting the scope you can avoid unnecessary serverless hits to improve performance and reduce cost.
-
Navigate to your property in Control Center.
-
Click Edit.
-
Click + Rules.
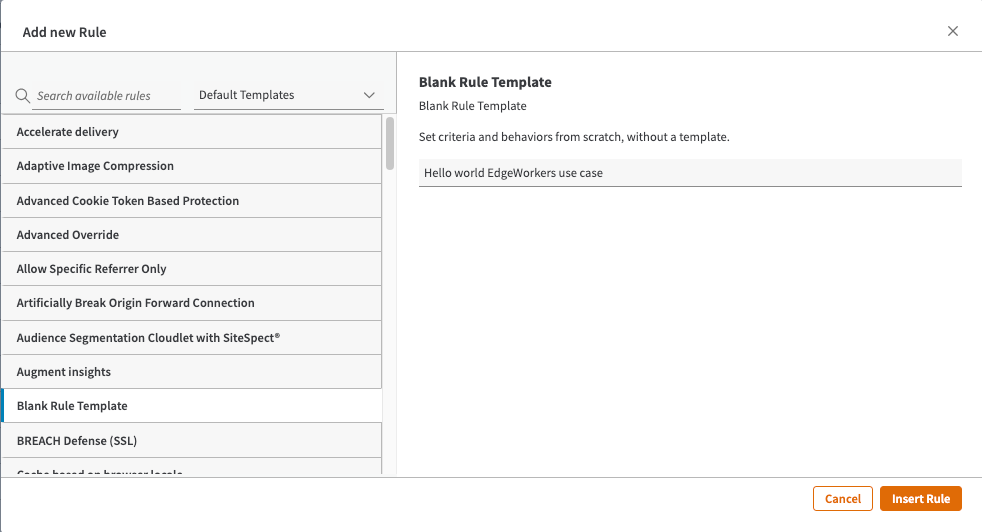
-
Enter a name for your rule and click the Insert Rule button.
-
Click the + Match button to add a
match criteria
andscope
.The criteria below only executes the EdgeWorkers function when the path matches
locationBasedRedirect
.
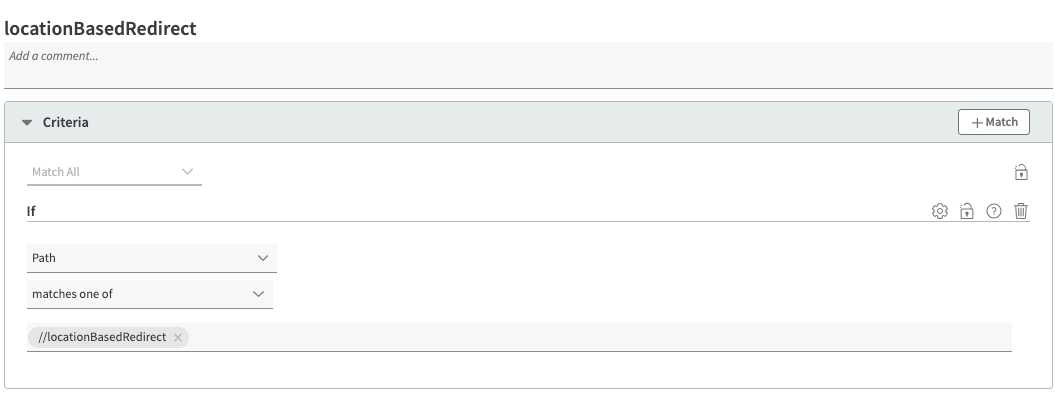
- Next, click the + Behavior button and select standard property behavior,
- Search for
EdgeWorkers
in available behaviors.
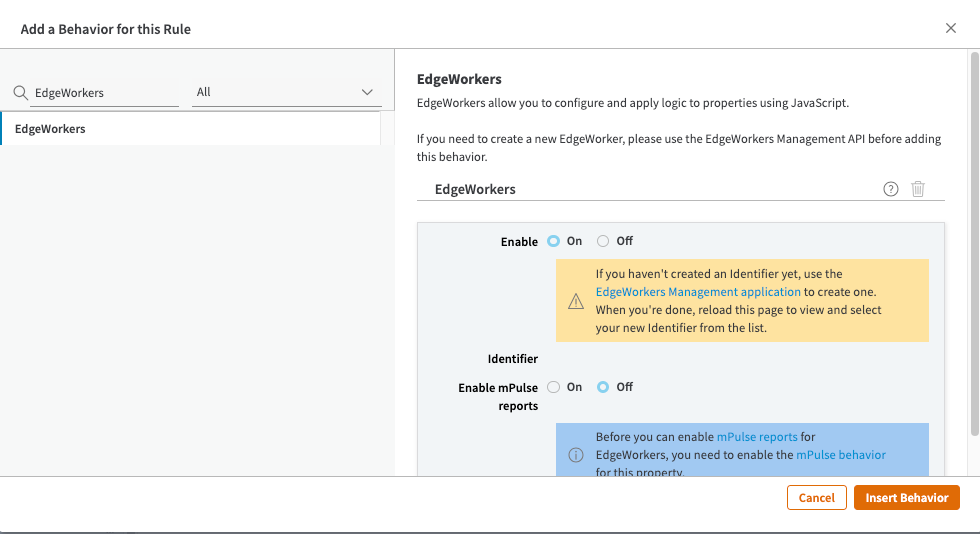
- Change the setting to On.
- Click Insert Behavior.
- Select the EdgeWorker ID that you created in step 1 from the list.
- Save your property.
- Click the Activate tab.
- Click the Activate on Staging button.
3. Create the code bundle
To create a code bundle you need a main.js
source file and a bundle.json
manifest file.
-
Create a folder on your computer for the code bundle files.
-
Go to the EdgeWorkers GitHub repository and download the
main.js
andbundle.json
files from the redirect- geo project or create them using the values below.
This JavaScript sample is commented to help you understand the logic behind the use case and code.
/*
(c) Copyright 2023 Akamai Technologies, Inc. Licensed under Apache 2 license.
Version: 0.1
Purpose: Using EdgeScape geo data, redirect user to country specific content
Repo: https://github.com/akamai/edgeworkers-examples/tree/master/redirect-geo
*/
//Define the top level domain mapping for Canada, the U.S., and UK.
const tldMap = {
CA: '.ca',
GB: '.co.uk',
US: '.com'
};
//Implement onClient request as the first event before caching occurs.
export function onClientRequest (request) {
//Create a subdomain from the host of the incoming request.
const subDomain = request.host.split('.')[0];
//Create a domain from the host of the incoming request.
const domain = request.host.split('.')[1];
//Determine the top level domain based on the request's country of origin.
let tld = tldMap[request.userLocation.country];
//Set the default domain to .com.
if (tld === undefined) {
tld = '.com';
}
//Build up new domain based on the location of the request. For example, .ca for Canada.
const redirectDomain = subDomain + '.' + domain + tld;
//Check the incoming domain to see if the incoming domain is different from the built up domain.
if (request.host !== redirectDomain) {
//Redirect to new host. If a redirect is required, return the location header with the new domain in the response.
request.respondWith(302, {
Location: [request.scheme + '://' + redirectDomain + request.url]
}, '');
}
}
- Create the
bundle.json
manifest file.
{
"edgeworker-version": "1 ",
"description" : "Perform redirect"
}
- Compress the files into a code bundle.
tar -czvf filename.tgz main.js bundle.json
4. Deploy the code bundle
To deploy the code bundle you need to create an EdgeWorker version.
-
Go to ☰ > CDN > EdgeWorkers.
-
From the EdgeWorkers IDs page, select the EdgeWorker ID that you just created.
-
Click the Create version button.
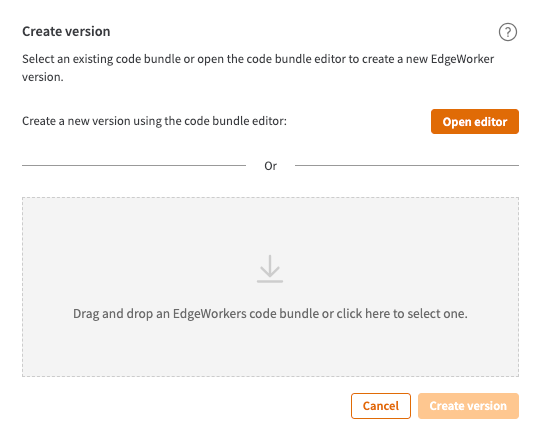
- Drag and drop the code bundle onto the window and click the Create version button.
5. Activate the EdgeWorker version
-
From the EdgeWorkers versions page, select your EdgeWorker ID.
Only the EdgeWorkers you have permission to access appear in the list.
-
Click the Activate version button.
-
Select the EdgeWorker version that you want to activate.
-
Select the Staging network to test your code sample.
6. Test the EdgeWorkers function
You can use a curl request, resolved to staging, to exercise the EdgeWorkers function.
For more information on how to resolve an IP address refer to the Getting Started for HTTPS Properties documentation.
-
Use this curl request to test the
locationBasedRedirect
EdgeWorkers function.Replace [akamai staging name] with the staging Edge hostname of your website. The staging Edge hostname uses the .edgekey-staging.net domain.
curl https://[your website name]/redirectGeo --connect-to ::[akamai staging name] -H "Pragma: akamai-x-ew-debug" -sD - -sD -
- The curl request should produce output similar the following.
HTTP/2 302
location: https://[your website name]/redirectGeo
content-type: text/html
content-length: 0
expires: Wed, 07 Jun 2023 15:18:43 GMT
cache-control: max-age=0, no-cache, no-store
pragma: no-cache
date: Wed, 07 Jun 2023 15:18:43 GMT
server-timing: cdn-cache; desc=HIT
server-timing: edge; dur=1
x-akamai-edgeworker-onclientresponse-info: ew=9xx v:Demo_RedirectGeo; status=UnimplementedEventHandler
x-akamai-edgeworker-onclientrequest-info: ew=9xx v0.9.2:Demo_RedirectGeo; status=Success
server-timing: ak_p; desc="1686151123657_381190694_44213894_27_8634_24_54_15";dur=1
Updated 3 months ago